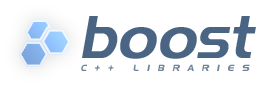
dynamic_bitset<Block, Allocator>
目录
描述
该dynamic_bitset类表示一组位。 它通过operator[]提供对各个位值的访问,并提供可以应用于内置整数的所有按位运算符,例如operator&和operator<<。 集合中的位数在运行时通过类的构造函数的参数指定。dynamic_bitset.
该dynamic_bitset类几乎与 std::bitset 类相同。 区别在于dynamic_bitset(位数)在运行时在构造一个dynamic_bitset对象期间指定,而std::bitset的大小在编译时通过整数模板参数指定。
旨在解决的主要问题是表示有限集的子集。 每个位表示有限集的元素是否在子集中。 因此,dynamic_bitset旨在解决的主要问题是表示有限集的子集。 每个位表示有限集的元素是否在子集中。 因此,的按位运算,例如dynamic_bitset,例如operator&和operator|,对应于集合运算,例如交集和并集。
概要
namespace boost { template <typename Block, typename Allocator> class dynamic_bitset { public: typedef Block block_type; typedef Allocator allocator_type; typedef implementation-defined size_type; static const int bits_per_block = implementation-defined; static const size_type npos = implementation-defined; class reference { void operator&(); // not defined public: // An automatically generated copy constructor. reference& operator=(bool value); reference& operator=(const reference& rhs); reference& operator|=(bool value); reference& operator&=(bool value); reference& operator^=(bool value); reference& operator-=(bool value); bool operator~() const; operator bool() const; reference& flip(); }; typedef bool const_reference; dynamic_bitset(); explicit dynamic_bitset(const Allocator& alloc); explicit dynamic_bitset(size_type num_bits, unsigned long value = 0, const Allocator& alloc = Allocator()); template <typename CharT, typename Traits, typename Alloc> explicit dynamic_bitset(const std::basic_string<CharT, Traits, Alloc>& s, typename std::basic_string<CharT, Traits, Alloc>::size_type pos = 0, typename std::basic_string<CharT, Traits, Alloc>::size_type n = std::basic_string<CharT, Traits, Alloc>::npos, const Allocator& alloc = Allocator()); template <typename BlockInputIterator> dynamic_bitset(BlockInputIterator first, BlockInputIterator last, const Allocator& alloc = Allocator()); dynamic_bitset(const dynamic_bitset& b); dynamic_bitset(dynamic_bitset&& b); void swap(dynamic_bitset& b); dynamic_bitset& operator=(const dynamic_bitset& b); dynamic_bitset& operator=(dynamic_bitset&& b); allocator_type get_allocator() const; void resize(size_type num_bits, bool value = false); void clear(); void pop_back(); void push_back(bool bit); void append(Block block); template <typename BlockInputIterator> void append(BlockInputIterator first, BlockInputIterator last); dynamic_bitset& operator&=(const dynamic_bitset& b); dynamic_bitset& operator|=(const dynamic_bitset& b); dynamic_bitset& operator^=(const dynamic_bitset& b); dynamic_bitset& operator-=(const dynamic_bitset& b); dynamic_bitset& operator<<=(size_type n); dynamic_bitset& operator>>=(size_type n); dynamic_bitset operator<<(size_type n) const; dynamic_bitset operator>>(size_type n) const; dynamic_bitset& set(size_type n, size_type len, bool val); dynamic_bitset& set(size_type n, bool val = true); dynamic_bitset& set(); dynamic_bitset& reset(size_type n, size_type len); dynamic_bitset& reset(size_type n); dynamic_bitset& reset(); dynamic_bitset& flip(size_type n, size_type len); dynamic_bitset& flip(size_type n); dynamic_bitset& flip(); reference at(size_type n); bool at(size_type n) const; bool test(size_type n) const; bool test_set(size_type n, bool val = true); bool all() const; bool any() const; bool none() const; dynamic_bitset operator~() const; size_type count() const noexcept; reference operator[](size_type pos); bool operator[](size_type pos) const; unsigned long to_ulong() const; size_type size() const noexcept; size_type num_blocks() const noexcept; size_type max_size() const noexcept; bool empty() const noexcept; size_type capacity() const noexcept; void reserve(size_type num_bits); void shrink_to_fit(); bool is_subset_of(const dynamic_bitset& a) const; bool is_proper_subset_of(const dynamic_bitset& a) const; bool intersects(const dynamic_bitset& a) const; size_type find_first() const; size_type find_first(size_type pos) const; size_type find_next(size_type pos) const; }; template <typename B, typename A> bool operator==(const dynamic_bitset<B, A>& a, const dynamic_bitset<B, A>& b); template <typename Block, typename Allocator> bool operator!=(const dynamic_bitset<Block, Allocator>& a, const dynamic_bitset<Block, Allocator>& b); template <typename B, typename A> bool operator<(const dynamic_bitset<B, A>& a, const dynamic_bitset<B, A>& b); template <typename Block, typename Allocator> bool operator<=(const dynamic_bitset<Block, Allocator>& a, const dynamic_bitset<Block, Allocator>& b); template <typename Block, typename Allocator> bool operator>(const dynamic_bitset<Block, Allocator>& a, const dynamic_bitset<Block, Allocator>& b); template <typename Block, typename Allocator> bool operator>=(const dynamic_bitset<Block, Allocator>& a, const dynamic_bitset<Block, Allocator>& b); template <typename Block, typename Allocator> dynamic_bitset<Block, Allocator> operator&(const dynamic_bitset<Block, Allocator>& b1, const dynamic_bitset<Block, Allocator>& b2); template <typename Block, typename Allocator> dynamic_bitset<Block, Allocator> operator|(const dynamic_bitset<Block, Allocator>& b1, const dynamic_bitset<Block, Allocator>& b2); template <typename Block, typename Allocator> dynamic_bitset<Block, Allocator> operator^(const dynamic_bitset<Block, Allocator>& b1, const dynamic_bitset<Block, Allocator>& b2); template <typename Block, typename Allocator> dynamic_bitset<Block, Allocator> operator-(const dynamic_bitset<Block, Allocator>& b1, const dynamic_bitset<Block, Allocator>& b2); template <typename Block, typename Allocator, typename CharT, typename Alloc> void to_string(const dynamic_bitset<Block, Allocator>& b, std::basic_string<CharT, Alloc>& s); template <typename Block, typename Allocator, typename BlockOutputIterator> void to_block_range(const dynamic_bitset<Block, Allocator>& b, BlockOutputIterator result); template <typename CharT, typename Traits, typename Block, typename Allocator> std::basic_ostream<CharT, Traits>& operator<<(std::basic_ostream<CharT, Traits>& os, const dynamic_bitset<Block, Allocator>& b); template <typename CharT, typename Traits, typename Block, typename Allocator> std::basic_istream<CharT, Traits>& operator>>(std::basic_istream<CharT, Traits>& is, dynamic_bitset<Block, Allocator>& b); } // namespace boost
定义
每个位表示布尔值 true 或 false(1 或 0)。 设置位是将它赋值为 1。 清除或重置位是将它赋值为 0。 翻转位是将值更改为 1(如果它是 0)和 0(如果它是 1)。 每个位都有一个非负位置。 一个位集合x包含x.size()位,每个位在以下范围内分配唯一的position[0,x.size())。 位置 0 处的位称为最低有效位,位置处的位size() - 1是最高有效位。 当从dynamic_bitset转换为或从 unsigned longn时,位置处的位i的位集合具有与以下相同的值(n >> i) & 1.
示例
示例 1 (设置和读取一些位)
示例 2 (从整数创建一些位集合)
示例 3 (执行输入/输出和一些按位运算)。
原理
dynamic_bitset不是一个 容器,并且由于以下原因不提供迭代器
- 具有代理的容器引用类型不能满足 C++ 标准中指定的容器要求(除非采用奇怪的迭代器语义)。std::vector<bool>有一个代理引用类型,并且不满足容器要求,因此导致了很多问题。 一个常见的问题是当人们尝试使用来自的迭代器时std::vector<bool>与标准算法(如std::search。 的std::search要求说迭代器必须是 Forward Iterator,但std::vector<bool>::iterator由于代理引用,不满足此要求。 根据实现,由于这种误用,它们可能是也可能不是编译错误,甚至运行时错误。 有关该问题的进一步讨论,请参阅 Scott Meyers 的 Effective STL)。 所以dynamic_bitset试图通过不假装是容器来避免这些问题。
有些人更喜欢使用名称“toggle”而不是“flip”。 选择名称“flip”是因为这是 std::bitset 中使用的名称。 事实上,大多数函数的名称dynamic_bitset都是为此原因而选择的。
dynamic_bitset当违反前提条件时不会抛出异常(如std::bitset)。 相反assert被使用。 有关解释,请参阅 错误和异常处理 的指南。
头文件
类dynamic_bitset在头文件 boost/dynamic_bitset.hpp 中定义。 此外,还有dynamic_bitset在头文件 boost/dynamic_bitset_fwd.hpp 中的前向声明。
模板参数
参数 | 描述 | 默认值 |
---|---|---|
Block | 存储位的整数类型。 | unsigned long |
Allocator | 用于所有内部内存管理的分配器类型。 | std::allocator<Block> |
概念模型
Assignable, Default Constructible, Equality Comparable, LessThan Comparable。类型要求
Block是一个无符号整数类型。Allocator满足分配器的标准要求。公共基类
无。嵌套类型名称
dynamic_bitset::reference
一个代理类,充当对单个位的引用。 它包含赋值运算符,转换为bool,一个operator~,以及一个成员函数flip。 它仅作为的辅助类存在dynamic_bitset的operator[]。 下表描述了对引用类型的有效操作。 假设b是的实例dynamic_bitset, i, j是size_type并且在范围内[0,b.size())。 另请注意,当我们写b[i]时,我们指的是类型的对象引用从初始化b[i]。 变量xxbool.
是 | 表达式 |
---|---|
x = b[i] | 分配的第 i 位bbx. |
(bool)b[i] | 返回的第 i 位b. |
b[i] = x | 设置的第 i 位bbx为的值,并返回b[i]. |
b[i] |= x | 或的第 i 位bbx为的值,并返回b[i]. |
以及的值 | b[i] &= xbbx为的值,并返回b[i]. |
与的第 i 位 | b[i] ^= xbbx为的值,并返回b[i]. |
异或的第 i 位 | b[i] -= x如果x==trueb,清除的第 i 位b[i]. |
b | 设置的第 i 位b。 返回b为的值,并返回b[i]. |
b[i] = b[j] | 或的第 i 位b为第 j 位的值b为的值,并返回b[i]. |
b[i] |= b[j] | b[i] &= xb为第 j 位的值b为的值,并返回b[i]. |
与第 j 位 | b[i] ^= xb为第 j 位的值b为的值,并返回b[i]. |
b[i] &= b[j] | b[i] ^= b[j]bb[i] -= b[j]b,清除的第 i 位b[i]. |
如果第 j 位 | bbbx. |
被设置,清除的第 i 位 | x = ~b[i]b. |
分配的第 i 位的相反值 | (bool)~b[i]b为的值,并返回b[i]. |
dynamic_bitset::const_reference返回的第 i 位的相反值bool.
dynamic_bitset::size_typeb[i].flip()
dynamic_bitset::block_type翻转的第 i 位Block.
dynamic_bitset::allocator_type;翻转的第 i 位Allocator.
公共数据成员
dynamic_bitset::bits_per_block类型BlockThe unsigned integer type for representing the size of the bit set.与类型.
dynamic_bitset::nposThe number of bits the typesize_type.
构造函数
dynamic_bitset()用于表示值,不包括任何填充位。 在数值上等于Allocator.
std::numeric_limits<Block>::digits 的最大值.
效果: 构造大小为零的位集。 此位集的分配器是类型为默认构造的对象Allocator后置条件
this->size() == 0
dynamic_bitset(const Allocator& alloc)抛出: 除非的默认构造函数Allocator抛出异常,否则不抛出任何异常。(Default Constructible 要求。)效果: 构造大小为零的位集。 的副本
std::numeric_limits<Block>::digits 的最大值.
alloc
dynamic_bitset(size_type num_bits, unsigned long value = 0, const Allocator& alloc = Allocator())对象将用于后续的位集操作,例如resize以分配内存。抛出: 无。效果: 从整数构造位集。 前M位被初始化为中相应的位Allocator抛出异常,否则不抛出任何异常。(Default Constructible 要求。)value
,所有其他位(如果有)都初始化为零(其中
M = min(num_bits, std::numeric_limits<unsigned long>::digits)
std::numeric_limits<Block>::digits
- )。 的副本
- alloci以分配内存。 请注意,例如,以下dynamic_bitset b<>( 16, 7 );, 将匹配 来自迭代器范围的构造函数(不是这个),但底层实现仍然会“做正确的事情”,并从值 7 构造一个 16 位的位集。.
- alloci以分配内存。 请注意,例如,以下后置条件, this->size() == num_bits.
dynamic_bitset(const dynamic_bitset& x)(*this)[i] == (value >> i) & 1x对于所有x.
ii以分配内存。 请注意,例如,以下[0,x.size()), 在范围内.
对于所有i在范围内[0,M)).
[M,num_bits)
dynamic_bitset(dynamic_bitset&& x)(*this)[i] == falsex抛出: 如果内存耗尽,则会发生分配错误(xstd::bad_allocx.
ii以分配内存。 请注意,例如,以下[0,x.size()), 在范围内.
对于所有i在范围内[0,M)).
template <typename BlockInputIterator> explicit dynamic_bitset(BlockInputIterator first, BlockInputIterator last, const Allocator& alloc = Allocator());如果
- Allocator=std::allocator)。效果: 构造一个位集,该位集是的副本unsigned longx。 此位集的分配器是的分配器的副本x
。// b is constructed as if by calling the constructor // // dynamic_bitset(size_type num_bits, // unsigned long value = 0, // const Allocator& alloc = Allocator()) // // with arguments // // static_cast<dynamic_bitset<unsigned short>::size_type>(8), // 7, // Allocator() // dynamic_bitset<unsigned short> b(8, 7);
后置条件: 对于所有
i(*this)[i] == x[i](Assignable 要求。)效果: 构造一个与位集相同的位集x,同时使用来自的资源x。 此位集的分配器是从中的分配器移动的x效果: 构造一个与位集相同的位集效果dynamic_bitset如果使用类型调用此构造函数
- BlockInputIterator实际上是整数类型,则库的行为就像是从构造函数调用一样来自整数的构造函数被调用,参数为static_cast<size_type>(first), last and alloc,按该顺序排列。示例b注意在编写时(2008 年 10 月),这与 库问题 438 的拟议解决方案一致。 这是一个 post C++03 更改,目前在C++0xb的工作文件中。非正式地说,关于的关键变化C++03是删除static_cast在第二个参数上,以及关于模板构造函数何时应具有与 (size, value) 构造函数相同的效果的更大余地:仅当InputIteratori是整数类型,在C++03).
中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 )。Boost.DynamicBitset,我们将自己限制在这两个更改中的第一个。否则(即,如果模板参数不是整数类型),则在条件下的构造Block.
对于所有i在范围内[0,M)).
template<typename Char, typename Traits, typename Alloc> explicit dynamic_bitset(const std::basic_string<Char,Traits,Alloc>& s, typename std::basic_string<CharT, Traits, Alloc>::size_type pos = 0, typename std::basic_string<CharT, Traits, Alloc>::size_type n = std::basic_string<Char,Traits,Alloc>::npos, const Allocator& alloc = Allocator())requires 子句——一个基于块范围的位集。 让*first0是块号 0,1.
*++firstresize块号 1,等等。 块号b用于初始化 position 范围内的 dynamic_bitset 的位[b*bits_per_block, (b+1)*bits_per_block)。 对于每个块号bb,值为bvali,位(bval >> i) & 1对应于位置处的位(b * bits_per_block + i).
在位集中(其中i在范围内[0,M)).
析构函数
~dynamic_bitset()i
alloc
遍历范围
void swap(dynamic_bitset& b);[0, bits_per_block)bRequires
InputIteratorb必须是整数类型或 Input Iterator 的模型,其bvalue_type
alloc
dynamic_bitset& operator=(const dynamic_bitset& x)与类型x.
ii以分配内存。 请注意,例如,以下[0,x.size()), 在范围内.
Block 相同。.
alloc
[M,num_bits)
dynamic_bitset& operator=(dynamic_bitset&& x)Preconditionx抛出: 如果内存耗尽,则会发生分配错误(x.
ii以分配内存。 请注意,例如,以下[0,x.size()), 在范围内.
Block 相同。.
对于所有i在范围内[0,M)).
allocator_type get_allocator() const;pos <= s.size()相同。.
void resize(size_type num_bits, bool value = false);并且用于初始化位的字符必须是'0'或'1'效果: 从 0 和 1 的字符串构造位集。 前M位被初始化为中相应的字符s,其中抛出: 无。或M = min(s.size() - pos, n)效果: 从 0 和 1 的字符串构造位集。 前。 请注意,中的最高字符位置s
std::numeric_limits<Block>::digits )。 的副本.
对于所有i在范围内[0,M)).
void clear(),而不是最低,对应于最低有效位。 也就是说,字符位置
alloc
void pop_back();requires pos + M - 1 - i.
对应于位
alloc
void push_back(bool value);i抛出: 无。.
对于所有i在范围内[0,M)).
void append(Block value);。 所以,例如,抛出: 无。dynamic_bitset(string("1101"))与相同dynamic_bitset(13ul)b抛出: 如果内存耗尽,则会发生分配错误(i以分配内存。 请注意,例如,以下std::bad_alloc时,位置处的位如果Allocator=std::allocator)。.
对于所有i在范围内[0,M)).
template <typename BlockInputIterator> void append(BlockInputIterator first, BlockInputIterator last);效果: 释放与此位集关联的内存并销毁位集对象本身。
for (; first != last; ++first) append(*first);成员函数)。效果: 此位集和位集的内容,我们将自己限制在这两个更改中的第一个。rhsBlock.
对于所有i在范围内[0,M)).
dynamic_bitset& operator&=(const dynamic_bitset& rhs)中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 被交换。.
后置条件: 此位集等于原始的rhs,并且
for (size_type i = 0; i != this->size(); ++i) (*this)[i] = (*this)[i] & rhs[i];Block 相同。.
alloc
dynamic_bitset& operator|=(const dynamic_bitset& rhs)中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 被交换。.
rhsrhs,并且
for (size_type i = 0; i != this->size(); ++i) (*this)[i] = (*this)[i] | rhs[i];Block 相同。.
alloc
dynamic_bitset& operator^=(const dynamic_bitset& rhs)中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 被交换。.
等于此位集的先前版本。rhs,并且
for (size_type i = 0; i != this->size(); ++i) (*this)[i] = (*this)[i] ^ rhs[i];Block 相同。.
alloc
dynamic_bitset& operator-=(const dynamic_bitset& rhs)中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 被交换。.
效果: 此位集成为位集的副本rhsx
for (size_type i = 0; i != this->size(); ++i) (*this)[i] = (*this)[i] && !rhs[i];Block 相同。.
alloc
dynamic_bitset& operator<<=(size_type n)返回n*this效果: 此位集变得与位集相同x
Block 相同。.
alloc
dynamic_bitset& operator>>=(size_type n)返回: 用于构造的分配器对象的副本n效果: 将位集的位数更改为num_bits。 如果num_bits > size()x
Block 相同。.
alloc
dynamic_bitset operator<<(size_type n) const,则范围内的位相同。[0,size())n保持不变,并且中的位效果: 此位集变得与位集相同[size(),num_bits)
对于所有i在范围内[0,M)).
dynamic_bitset operator>>(size_type n) const,则范围内的位相同。都设置为n保持不变,并且中的位num_bits > size()[size(),num_bits)
对于所有i在范围内[0,M)).
dynamic_bitset& set()zero
Block 相同。
alloc
dynamic_bitset& flip()。 如果
Block 相同。
alloc
dynamic_bitset operator~() const,则范围内的位相同。num_bits < size()
对于所有i在范围内[0,M)).
dynamic_bitset& reset(),则范围内的位
Block 相同。
alloc
dynamic_bitset& set(size_type n, size_type len, bool val);requires [0,num_bits).
保持不变(剩余的位将被丢弃)。nb效果: 位集的大小变为零。后置条件:this->empty()前置条件:!this->empty()效果: 将位集的大小减小 1。this->empty()前置条件:效果: 将位集的大小增加 1,并将新的最高有效位的值设置为.
Block 相同。
dynamic_bitset& set(size_type n, bool val = true)requires zero.
效果: 将中的位附加到该位集(附加到最高有效端)。 这将位集的大小增加n在范围内this->empty()前置条件:!this->empty()valuen在范围内this->empty()前置条件:效果: 将位集的大小增加 1,并将新的最高有效位的值设置为.
Block 相同。
dynamic_bitset& reset(size_type n, size_type len);requires [0,num_bits).
到位集(附加到最高有效端)。 这将位集的大小增加nb效果: 位集的大小变为零。bits_per_block
Block 相同。
dynamic_bitset& reset(size_type n)requires zero.
。 让n.
Block 相同。
dynamic_bitset& flip(size_type n, size_type len)requires [0,num_bits).
snb效果: 位集的大小变为零。bits_per_block
Block 相同。
dynamic_bitset& flip(size_type n)requires zero.
是位集的旧大小,然后对于n.
Block 相同。
size_type size() consti
alloc
size_type num_blocks() const在
alloc
size_type max_size() const;[0,bits_per_block)dynamic_bitset,位相同。(*this)[dynamic_bitset(s + i)]被设置为((value >> i) & 1)效果: 此函数提供与以下代码相同的最终结果,但通常效率更高。
要求: 类型
bool empty() const;Block !this->empty()在范围内的最大值, 效果: 将位集的大小增加 1,并将新的最高有效位的值设置为InputIterator必须是 Input Iterator 的模型,并且value_type
size_type capacity() const;必须与类型相同。Block
alloc
void reserve(size_type num_bits);相同。
后置条件:
this->size() == rhs.size() 效果: 按位与所有位.
对于所有i在范围内[0,M)).
void shrink_to_fit();rhs
后置条件:
对于所有i在范围内[0,M)).
size_type count() const与此位集中的位。 这等效于
alloc
bool all() constBlock !this->empty()operator&=(rhs)效果: 按位或所有位rhs效果: 将位集的大小增加 1,并将新的最高有效位的值设置为.
alloc
bool any() constBlock !this->empty()与此位集中的位。 这等效于效果: 将位集的大小增加 1,并将新的最高有效位的值设置为.
alloc
bool none() constBlock !this->empty()operator|=(rhs)效果: 将位集的大小增加 1,并将新的最高有效位的值设置为.
alloc
reference at(size_type n)requires zero.
效果: 按位异或所有位rhs.
与此位集中的位。 这等效于 operator^=(rhs)效果: 计算此位集与之间的集合差nrhs
bool at(size_type n) constrequires zero.
效果: 按位异或所有位rhs.
与此位集中的位。 这等效于 operator^=(rhs)效果: 计算此位集与之间的集合差nrhs
bool test(size_type n) constrequires zero.
Block !this->empty()位集。 这等效于noperator-=(rhs)效果: 将位集的大小增加 1,并将新的最高有效位的值设置为效果: 将此位集中的位向左移动nn
bool test_set(size_type n, bool val = true)requires zero.
效果: 将中的位附加到该位集(附加到最高有效端)。 这将位集的大小增加n在范围内this->empty()前置条件:!this->empty()valuen在范围内this->empty()前置条件:效果: 将位集的大小增加 1,并将新的最高有效位的值设置为.
Block !this->empty()位。 对于位集中的每个位,位置 pos 处的位采用位置处位的先前值npos - n效果: 将位集的大小增加 1,并将新的最高有效位的值设置为效果: 将此位集中的位向左移动nn
reference operator[](size_type n)requires zero.
,如果不存在此类位,则为零。引用效果: 将此位集中的位向右移动nn引用位。 对于位集中的每个位,位置处的位boolposoperator[]采用位的前一个值pos + n和返回: 的副本b向左移动.
bool operator[](size_type n) constrequires zero.
效果: 按位异或所有位n.
unsigned long to_ulong() const位。 对于返回的位集中的每个位,位置 pos 处的位采用位置处位的值相同。.
与此位集中的位。 这等效于 pos + n此位集,如果不存在此类位,则为零。unsigned long返回: 的副本相同。b向右移动.
bool is_subset_of(const dynamic_bitset& a) const中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 n
位。效果: 将此位集中的每个位设置为 1。效果: 翻转此位集中每个位的值。效果: 将此位集中的每个位设置为 1。返回: 位集
alloc
bool is_proper_subset_of(const dynamic_bitset& a) const中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 n
b效果: 将此位集中的每个位设置为 1。效果: 翻转此位集中每个位的值。效果: 将此位集中的每个位设置为 1。,及其所有位都被翻转。效果: 清除此位集中的每个位。前置条件:
alloc
bool intersects(const dynamic_bitset& a) const中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 n
n + len < this->size()效果: 将此位集中的每个位设置为 1。效果: 设置从索引的每个位效果: 将此位集中的每个位设置为 1。返回: 位集
alloc
size_type find_first() const;ni到in + len - 1(包括)如果在范围内相同。val
size_type find_first(size_type pos) const;ni是true到in + len - 1(包括)如果,如果是
size_type find_next(size_type pos) const;nifalsenum_bits到in + len - 1(包括)如果,如果是
bool operator==(const dynamic_bitset& rhs) constBlock !this->empty()在范围内被交换。,则清除它们。i以分配内存。 请注意,例如,以下前置条件:, n < this->size()效果: 设置位效果: 将位集的大小增加 1,并将新的最高有效位的值设置为.
alloc
n
bool operator!=(const dynamic_bitset& rhs) constBlock ,并清除位
alloc
n
bool operator<(const dynamic_bitset& rhs) constBlock !this->empty()nrhs效果: 清除从索引的每个位效果: 将位集的大小增加 1,并将新的最高有效位的值设置为n
alloc
(包括)。
bool operator>(const dynamic_bitset& rhs) constBlock 效果: 清除位
alloc
(包括)。
bool operator<=(const dynamic_bitset& rhs) constBlock n
alloc
(包括)。
bool operator>=(const dynamic_bitset& rhs) constBlock 效果: 翻转从索引的每个位
alloc
(包括)。
n
dynamic_bitset operator&(const dynamic_bitset& a, const dynamic_bitset& b)中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 到
n + len - 1效果: 将此位集中的每个位设置为 1。和b.
对于所有i在范围内[0,M)).
dynamic_bitset operator|(const dynamic_bitset& a, const dynamic_bitset& b)中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 到
(包括)。效果: 将此位集中的每个位设置为 1。和b.
对于所有i在范围内[0,M)).
dynamic_bitset operator^(const dynamic_bitset& a, const dynamic_bitset& b)中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 到
效果: 翻转位效果: 将此位集中的每个位设置为 1。和b.
对于所有i在范围内[0,M)).
dynamic_bitset operator-(const dynamic_bitset& a, const dynamic_bitset& b)中; 当它要么是整数类型,要么是实现可能检测为不可能成为输入迭代器的任何其他类型时,采用拟议的解决方案。 为了的目的 到
n效果: 将此位集中的每个位设置为 1。和b.
对于所有i在范围内[0,M)).
template <typename CharT, typename Alloc> void to_string(const dynamic_bitset<Block, Allocator>& b, std::basic_string<Char,Traits,Alloc>& s)返回: 此位集中的位数。b返回: 此位集中的块数。b返回: 的最大大小'1'dynamic_bitset'0'对象,具有与类型相同的对象ithis。 请注意,如果任何.
操作导致
size()超过max_size()
template <typename Block, typename Alloc, typename BlockOutputIterator> void to_block_range(const dynamic_bitset<Block, Alloc>& b, BlockOutputIterator result),则行为未定义。[当 lib 问题 197 关闭时,此函数的语义可能会略有变化]返回:std::bad_alloctrue如果此位集中设置了所有位,或者如果size() == 0非正式地说,关于的关键变化,否则返回是删除static_cast在第二个参数上,以及关于模板构造函数何时应具有与 (size, value) 构造函数相同的效果的更大余地:仅当false
。 注意:不要与混淆none(),它具有不同的语义。,我们将自己限制在这两个更改中的第一个。rhsBlock返回:true.
template <typename BlockIterator, typename Block, typename Alloc> void from_block_range(BlockIterator first, BlockIterator last, const dynamic_bitset<Block, Alloc>& b)如果此位集中设置了任何位,否则返回
。 注意:不要与混淆false返回:,我们将自己限制在这两个更改中的第一个。rhsBlocktruetrue.
template <typename Char, typename Traits, typename Block, typename Alloc> basic_ostream<Char, Traits>& operator<<(basic_ostream<Char, Traits>& os, const dynamic_bitset<Block, Alloc>& b)如果没有设置任何位,否则返回false返回: 与相同
std::basic_string<Char, Traits> s; boost::to_string(x, s): os << s;operator[](n)falseThrowsstd::out_of_range如果bn不在位集的范围内。返回:btrue如果位n被设置,并且falsei是位n, 是 0。返回:falsetruebbfalse如果位的前一个状态
n
与此位集中的位。 这等效于 被设置,并且false
template <typename Char, typename Traits, typename Block, typename Alloc> std::basic_istream<Char,Traits>& operator>>(std::basic_istream<Char,Traits>& is, dynamic_bitset<Block, Alloc>& b)否则。dynamic_bitset返回: 一个
定义
reference
- 到位n。 注意前置条件:reference
- 是一个代理类,具有赋值运算符和转换为
- n抛出:
- std::overflow_error
- 如果该值太大而无法在
unsigned long中表示,即如果b
在位置有任何非零位被设置,并且.
------
对于所有i在范围内[0,M)>= std::numeric_limits<unsigned long>::digits被设置,并且返回:
异常保证
truedynamic_bitset如果与先前版本的更改
this->size() == a.size()
- 和
- 返回: 如果此位集是位集的子集,则为 true
- a
- 。 也就是说,如果对于此位集中设置的每个位,位集中相应的位
a
- 也被设置,则返回 true。 否则此函数返回 false。
返回: 如果此位集是位集的真子集,则为 true
- a超过。 也就是说,它返回 true 如果dynamic_bitset(*this) < a((value >> i) & 1)和位集中相应的位超过a也被设置,并且如果this->count() < a.count()
。 否则此函数返回 false。
- 返回: 如果此位集和a, 相交,则为 true。 也就是说,如果在此位集中设置了一个位,使得位集中相应的位 , a, 也被设置,则返回 true。 否则此函数返回 false。, 返回: 最低索引 , ((value >> i) & 1) i
- ,例如位超过i
- 类引用dynamic_bitset::nposdynamic_bitset如果
- 没有设置位。引用.
另请参阅
std::bitset, 返回: 最低索引,致谢
i
,例如位 | i 被清除,或 |
npos | 如果 |
b | 没有清除位。 |
b | 返回: 最低索引 |
b | i |